Create blank scene
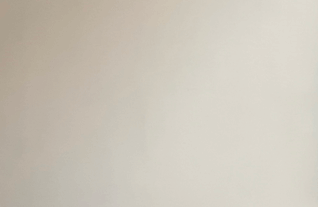
Description
We will create a completely blank scene, which when run will just show the camera view.
Whilst not an exciting step, it is a necessary one to check that the app is deploying to the phone correctly and we have done the prerequisites.
From here, we can start doing some cool stuff.
Video
No video yet
Code
using ARKit;
using Foundation;
using SceneKit;
using System;
using System.Linq;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
// Lesson: Create Blank Scene
this.sceneView = new ARSCNView();
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration
{
AutoFocusEnabled = true,
LightEstimationEnabled = true,
WorldAlignment = ARWorldAlignment.Gravity
}, ARSessionRunOptions.ResetTracking | ARSessionRunOptions.RemoveExistingAnchors);
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
}
Next Step : Show coordinate axis
After you have mastered this you should try Show coordinate axis