Show coordinate axis
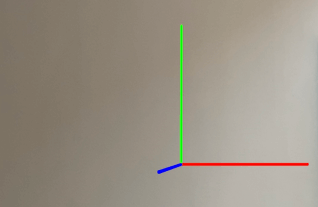
Description
We will show the built in co-ordinate guide in the scene. When the AR scene starts it places this at the World Origin which is where the camera was when the app was launched.
This is achieved by setting the ARSCNDebugOptions.ShowWorldOrigin
flag in the DebugOptions when creating the ARSCNView
.
This is useful for learning which way X, Y, and Z is in the scene.
Just remember..
- X = Left to Right. i.e Left is -X and Right is +X
- Y = Down to Up. i.e. Down is -Y and Up is +Y
- Z = Forwards to Backwards. i.e. Forwards is -Z and Backwards is +Z
So looking at the Coordinate helper when it is enabled.. Green is Y, Red is X and Blue is Z.
Video
No video yet
Code
using ARKit;
using System;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
// Lesson: Show world origin
this.sceneView = new ARSCNView
{
AutoenablesDefaultLighting = true,
DebugOptions = ARSCNDebugOptions.ShowWorldOrigin
};
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration
{
AutoFocusEnabled = true,
LightEstimationEnabled = true,
WorldAlignment = ARWorldAlignment.Gravity
}, ARSessionRunOptions.ResetTracking | ARSessionRunOptions.RemoveExistingAnchors);
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
}
Next Step : Show feature point detection
After you have mastered this you should try Show feature point detection