Show feature point detection
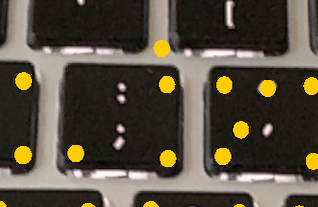
Description
We will use the built in ARSCNDebugOptions.ShowFeaturePoints
flag in the DebugOptions to show the feature points that are detected in the scene.
How well the feature points are detected depends on the type of surface the camera is looking at and the lighting conditions. Dark, featureless and reflective surfaces are difficult to identify feature points.
ARKit uses feature points to map and remember a scene.
Video
No video yet
Code
using ARKit;
using Foundation;
using SceneKit;
using System;
using System.Linq;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
// Lesson: Show feature points
this.sceneView = new ARSCNView
{
AutoenablesDefaultLighting = true,
DebugOptions = ARSCNDebugOptions.ShowFeaturePoints
};
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration
{
AutoFocusEnabled = true,
LightEstimationEnabled = true,
WorldAlignment = ARWorldAlignment.Gravity
}, ARSessionRunOptions.ResetTracking | ARSessionRunOptions.RemoveExistingAnchors);
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
}
Next Step : Show statistics
After you have mastered this you should try Show statistics