Remove item from scene
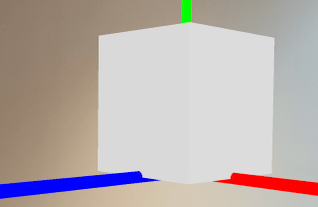
Description
We will add an item to the scene, then remove it when it has been touched.
Video
No video yet
Code
using ARKit;
using Foundation;
using SceneKit;
using System;
using System.Linq;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
this.sceneView = new ARSCNView
{
AutoenablesDefaultLighting = true,
DebugOptions = ARSCNDebugOptions.ShowFeaturePoints
| ARSCNDebugOptions.ShowWorldOrigin
};
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration
{
AutoFocusEnabled = true,
PlaneDetection = ARPlaneDetection.Horizontal,
LightEstimationEnabled = true,
WorldAlignment = ARWorldAlignment.GravityAndHeading
}, ARSessionRunOptions.ResetTracking | ARSessionRunOptions.RemoveExistingAnchors);
var size = 0.05f;
var cubeNode = new CubeNode(size, UIColor.White);
this.sceneView.Scene.RootNode.AddChildNode(cubeNode);
}
public override void TouchesEnded(NSSet touches, UIEvent evt)
{
base.TouchesEnded(touches, evt);
if (touches.AnyObject is UITouch touch)
{
var point = touch.LocationInView(this.sceneView);
var hitTestOptions = new SCNHitTestOptions();
var hits = this.sceneView.HitTest(point, hitTestOptions);
var hit = hits.FirstOrDefault();
if (hit == null)
return;
var node = hit.Node;
if (node == null)
return;
// Lesson - Remove node
node.RemoveFromParentNode();
}
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
public class CubeNode : SCNNode
{
public CubeNode(float size, UIColor color)
{
var rootNode = new SCNNode
{
Geometry = CreateGeometry(size, color),
Position = new SCNVector3(0, size / 2, 0)
};
AddChildNode(rootNode);
}
private static SCNGeometry CreateGeometry(float size, UIColor color)
{
var material = new SCNMaterial();
material.Diffuse.Contents = color;
var geometry = SCNBox.Create(size, size, size, 0);
geometry.Materials = new[] { material };
return geometry;
}
}
}
Next Step : Angle nodes with rotation
After you have mastered this you should try Angle nodes with rotation