Angle nodes with rotation
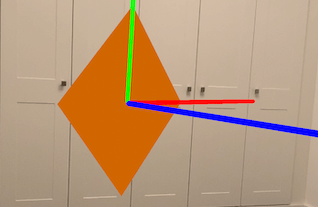
Description
We will learn how to angle nodes we have placed in the scene.
Video
No video yet
Code
using ARKit;
using SceneKit;
using System;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
this.sceneView = new ARSCNView
{
AutoenablesDefaultLighting = true,
DebugOptions = ARSCNDebugOptions.ShowWorldOrigin
};
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration
{
AutoFocusEnabled = true,
PlaneDetection = ARPlaneDetection.Horizontal,
LightEstimationEnabled = true,
WorldAlignment = ARWorldAlignment.GravityAndHeading
}, ARSessionRunOptions.ResetTracking | ARSessionRunOptions.RemoveExistingAnchors);
var width = 0.1f;
var length = 0.1f;
var planeNode = new PlaneNode(width, length, UIColor.Orange);
// Lesson: Rotate node
planeNode.Rotation = new SCNVector4(0, 0, 1, ConvertDegreesToRadians(45));
this.sceneView.Scene.RootNode.AddChildNode(planeNode);
}
public float ConvertDegreesToRadians(float angle)
{
return (float)(Math.PI / 180) * angle;
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
public class PlaneNode : SCNNode
{
public PlaneNode(float width, float length, UIColor color)
{
var rootNode = new SCNNode
{
Geometry = CreateGeometry(width, length, color)
};
AddChildNode(rootNode);
}
private static SCNGeometry CreateGeometry(float width, float length, UIColor color)
{
var material = new SCNMaterial();
material.Diffuse.Contents = color;
material.DoubleSided = true;
var geometry = SCNPlane.Create(width, length);
geometry.Materials = new[] { material };
return geometry;
}
}
}
Next Step : Front, back and sides
After you have mastered this you should try Front, back and sides