Sizes
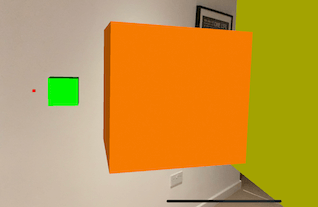
Description
In SceneKit, sizes are float data types, the size of 1f is equivalent to 1m. Therefore 0.1f is 10cm and 0.01f is 1cm.
Be careful not to make an object in your scene too big as you may not be able to see it (because you are effectively inside it!)
Video
No video yet
Code
using ARKit;
using SceneKit;
using System;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
this.sceneView = new ARSCNView
{
AutoenablesDefaultLighting = true
};
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration());
// 1cm
var cubeNode1 = new CubeNode(0.01f, UIColor.Red);
cubeNode1.Position = new SCNVector3(0, 0, 0);
// 10cm
var cubeNode2 = new CubeNode(0.1f, UIColor.Green);
cubeNode2.Position = new SCNVector3(0.1f, 0, 0);
// 50cm (0.5m)
var cubeNode3 = new CubeNode(0.5f, UIColor.Orange);
cubeNode3.Position = new SCNVector3(0.5f, 0, 0);
// 100cm (1m)
var cubeNode4 = new CubeNode(1f, UIColor.Yellow);
cubeNode4.Position = new SCNVector3(1.5f, 0, 0);
this.sceneView.Scene.RootNode.AddChildNode(cubeNode1);
this.sceneView.Scene.RootNode.AddChildNode(cubeNode2);
this.sceneView.Scene.RootNode.AddChildNode(cubeNode3);
this.sceneView.Scene.RootNode.AddChildNode(cubeNode4);
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
}
public class CubeNode : SCNNode
{
public CubeNode(float size, UIColor color)
{
var material = new SCNMaterial();
material.Diffuse.Contents = color;
var geometry = SCNBox.Create(size, size, size, 0);
geometry.Materials = new[] { material };
var rootNode = new SCNNode();
rootNode.Geometry = geometry;
AddChildNode(rootNode);
}
}
}
Next Step : Shapes
After you have mastered this you should try Shapes