Shapes
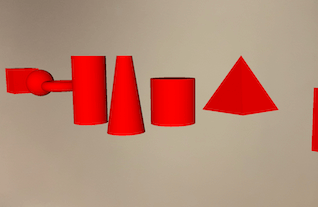
Description
There are a number of built in shapes (Geometries) you may wish to make use of.
Including Sphere, Box, Plane, Cylinder, Tube, Torus, Pyramid and Cone.
Video
No video yet
Code
using ARKit;
using SceneKit;
using System;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
this.sceneView = new ARSCNView
{
AutoenablesDefaultLighting = true
};
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
private SCNMaterial GetMaterial(UIColor color)
{
var material = new SCNMaterial();
material.Diffuse.Contents = color;
return material;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration
{
AutoFocusEnabled = true,
LightEstimationEnabled = true,
WorldAlignment = ARWorldAlignment.Gravity,
});
var size = 0.05f;
var zPosition = -0.8f;
var boxNode = new SCNNode();
boxNode.Geometry = SCNBox.Create(size, size, size, 0);
boxNode.Geometry.Materials = new SCNMaterial[] { GetMaterial(UIColor.Red) };
boxNode.Position = new SCNVector3(0, 0, zPosition);
var sphereNode = new SCNNode();
sphereNode.Geometry = SCNSphere.Create(size / 2);
sphereNode.Geometry.Materials = new SCNMaterial[] { GetMaterial(UIColor.Orange) };
sphereNode.Position = new SCNVector3(0.1f, 0, zPosition);
var torusNode = new SCNNode();
torusNode.Geometry = SCNTorus.Create(size / 2, size / 6);
torusNode.Geometry.Materials = new SCNMaterial[] { GetMaterial(UIColor.Yellow) };
torusNode.Position = new SCNVector3(0.2f, 0, zPosition);
var tubeNode = new SCNNode();
tubeNode.Geometry = SCNTube.Create(size / 2, (size / 2), size * 2);
tubeNode.Geometry.Materials = new SCNMaterial[] { GetMaterial(UIColor.Green) };
tubeNode.Position = new SCNVector3(0.3f, 0, zPosition);
var coneNode = new SCNNode();
coneNode.Geometry = SCNCone.Create(0.0001f, (size / 2), size * 2);
coneNode.Geometry.Materials = new SCNMaterial[] { GetMaterial(UIColor.Blue) };
coneNode.Position = new SCNVector3(0.4f, 0, zPosition);
var cylinderNode = new SCNNode();
cylinderNode.Geometry = SCNCylinder.Create(size / 2, size);
cylinderNode.Geometry.Materials = new SCNMaterial[] { GetMaterial(UIColor.Purple) };
cylinderNode.Position = new SCNVector3(0.5f, 0, zPosition);
var pyramidNode = new SCNNode();
pyramidNode.Geometry = SCNPyramid.Create(size, size, size);
pyramidNode.Geometry.Materials = new SCNMaterial[] { GetMaterial(UIColor.SystemPinkColor) };
pyramidNode.Position = new SCNVector3(0.6f, 0, zPosition);
var planeNode = new SCNNode();
planeNode.Geometry = SCNPlane.Create(size, size);
planeNode.Geometry.Materials = new SCNMaterial[] { GetMaterial(UIColor.Magenta) };
planeNode.Position = new SCNVector3(0.7f, 0, zPosition);
this.sceneView.Scene.RootNode.AddChildNode(boxNode);
this.sceneView.Scene.RootNode.AddChildNode(sphereNode);
this.sceneView.Scene.RootNode.AddChildNode(torusNode);
this.sceneView.Scene.RootNode.AddChildNode(tubeNode);
this.sceneView.Scene.RootNode.AddChildNode(coneNode);
this.sceneView.Scene.RootNode.AddChildNode(cylinderNode);
this.sceneView.Scene.RootNode.AddChildNode(pyramidNode);
this.sceneView.Scene.RootNode.AddChildNode(planeNode);
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
}
Next Step : Place text in scene
After you have mastered this you should try Place text in scene