Play movie
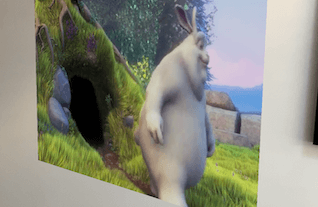
Description
We will take a local movie file (.mp4) and play it on a floating plane in the AR scene.
We do this by creating a SKVideoNode
, adding it to a SKScene
and set that as the material on the 2d plane.
Video
No video yet
Code
using ARKit;
using SceneKit;
using SpriteKit;
using System;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
this.sceneView = new ARSCNView
{
AutoenablesDefaultLighting = true
};
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration
{
AutoFocusEnabled = true,
LightEstimationEnabled = true,
WorldAlignment = ARWorldAlignment.Gravity
}, ARSessionRunOptions.ResetTracking | ARSessionRunOptions.RemoveExistingAnchors);
var videoNode = new SKVideoNode("Videos/big-buck-bunny-wide.mp4");
// Without this the video will be inverted upside down and back to front
videoNode.YScale = -1;
videoNode.Play();
var videoScene = new SKScene();
videoScene.Size = new CoreGraphics.CGSize(640, 360);
videoScene.ScaleMode = SKSceneScaleMode.AspectFill;
videoNode.Position = new CoreGraphics.CGPoint(videoScene.Size.Width / 2, videoScene.Size.Height / 2);
videoScene.AddChild(videoNode);
// These are set to be the same aspect ratio as the video itself (1.77 in this case)
var width = 0.5f;
var length = 0.28f;
var planeNode = new PlaneNode(width, length, new SCNVector3(0, 0, -0.5f), videoScene);
this.sceneView.Scene.RootNode.AddChildNode(planeNode);
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
public class PlaneNode : SCNNode
{
public PlaneNode(float width, float length, SCNVector3 position, SKScene videoScene)
{
var rootNode = new SCNNode
{
Geometry = CreateGeometry(width, length, videoScene),
Position = position
};
AddChildNode(rootNode);
}
private static SCNGeometry CreateGeometry(float width, float length, SKScene videoScene)
{
var material = new SCNMaterial();
material.Diffuse.Contents = videoScene;
material.DoubleSided = true;
var geometry = SCNPlane.Create(width, length);
geometry.Materials = new[] { material };
return geometry;
}
}
}
Next Step : Place 3d model in scene
After you have mastered this you should try Place 3d model in scene