Opacity
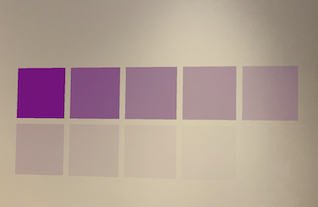
Description
We can control how transparent or opaque an element in our scene is using the .Opacity
property where 0.1f is 10% opaque and 1f is 100% opaque.
Opacity is a float and by default is 1f (100% opaque).
Video
No video yet
Code
using ARKit;
using SceneKit;
using System;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
this.sceneView = new ARSCNView
{
AutoenablesDefaultLighting = true
};
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration
{
AutoFocusEnabled = true,
PlaneDetection = ARPlaneDetection.Horizontal,
LightEstimationEnabled = true,
WorldAlignment = ARWorldAlignment.GravityAndHeading
}, ARSessionRunOptions.ResetTracking | ARSessionRunOptions.RemoveExistingAnchors);
var size = 0.09f;
var colour = UIColor.Purple;
var topRowY = 0.1f;
var bottomRowY = 0f;
this.sceneView.Scene.RootNode.AddChildNode(new PlaneNode(size, colour, new SCNVector3(-0.2f, topRowY, 0), 1.0f));
this.sceneView.Scene.RootNode.AddChildNode(new PlaneNode(size, colour, new SCNVector3(-0.1f, topRowY, 0), 0.9f));
this.sceneView.Scene.RootNode.AddChildNode(new PlaneNode(size, colour, new SCNVector3(0, topRowY, 0), 0.8f));
this.sceneView.Scene.RootNode.AddChildNode(new PlaneNode(size, colour, new SCNVector3(0.1f, topRowY, 0), 0.7f));
this.sceneView.Scene.RootNode.AddChildNode(new PlaneNode(size, colour, new SCNVector3(0.2f, topRowY, 0), 0.6f));
this.sceneView.Scene.RootNode.AddChildNode(new PlaneNode(size, colour, new SCNVector3(-0.2f, bottomRowY, 0), 0.5f));
this.sceneView.Scene.RootNode.AddChildNode(new PlaneNode(size, colour, new SCNVector3(-0.1f, bottomRowY, 0), 0.4f));
this.sceneView.Scene.RootNode.AddChildNode(new PlaneNode(size, colour, new SCNVector3(0f, bottomRowY, 0), 0.3f));
this.sceneView.Scene.RootNode.AddChildNode(new PlaneNode(size, colour, new SCNVector3(0.1f, bottomRowY, 0), 0.2f));
this.sceneView.Scene.RootNode.AddChildNode(new PlaneNode(size, colour, new SCNVector3(0.2f, bottomRowY, 0), 0.1f));
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
public class PlaneNode : SCNNode
{
public PlaneNode(float size, UIColor color, SCNVector3 position, float opacity)
{
var rootNode = new SCNNode
{
Geometry = CreateGeometry(size, color),
Position = position,
Opacity = opacity
};
AddChildNode(rootNode);
}
private static SCNGeometry CreateGeometry(float size, UIColor color)
{
var material = new SCNMaterial();
material.Diffuse.Contents = color;
var geometry = SCNPlane.Create(size, size);
geometry.Materials = new[] { material };
return geometry;
}
}
}
Next Step : Surface plane detection
After you have mastered this you should try Surface plane detection