Front, back and sides
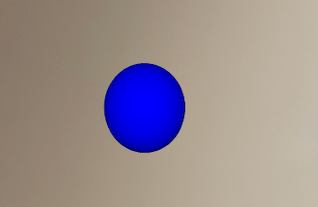
Description
We will place items in the scene, in front, behind and to each side of us. And also above and below us.
This is to practice the co-ordinate system and practice surrounding ourselves with things.
Video
No video yet
Code
using ARKit;
using SceneKit;
using System;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
this.sceneView = new ARSCNView
{
AutoenablesDefaultLighting = true,
DebugOptions = ARSCNDebugOptions.ShowFeaturePoints
| ARSCNDebugOptions.ShowWorldOrigin
};
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration
{
AutoFocusEnabled = true,
PlaneDetection = ARPlaneDetection.Horizontal,
LightEstimationEnabled = true,
WorldAlignment = ARWorldAlignment.GravityAndHeading
}, ARSessionRunOptions.ResetTracking | ARSessionRunOptions.RemoveExistingAnchors);
// Lesson - Front, back, sides, above and below
var size = 0.05f;
var distanceAway = 1f;
// Front
var cubeNodeFront = new SphereNode(size, UIColor.Yellow)
{
Position = new SCNVector3(distanceAway, 0, 0)
};
this.sceneView.Scene.RootNode.AddChildNode(cubeNodeFront);
// Back
var cubeNodeBack = new SphereNode(size, UIColor.Blue)
{
Position = new SCNVector3(-distanceAway, 0, 0)
};
this.sceneView.Scene.RootNode.AddChildNode(cubeNodeBack);
// Right
var cubeNodeRight = new SphereNode(size, UIColor.Red)
{
Position = new SCNVector3(0, 0, distanceAway)
};
this.sceneView.Scene.RootNode.AddChildNode(cubeNodeRight);
// Left
var cubeNodeLeft = new SphereNode(size, UIColor.Green)
{
Position = new SCNVector3(0, 0, -distanceAway)
};
this.sceneView.Scene.RootNode.AddChildNode(cubeNodeLeft);
// Above
var cubeNodeAbove = new SphereNode(size, UIColor.Orange)
{
Position = new SCNVector3(0, distanceAway, 0)
};
this.sceneView.Scene.RootNode.AddChildNode(cubeNodeAbove);
// Below
var cubeNodeBelow = new SphereNode(size, UIColor.Purple)
{
Position = new SCNVector3(0, -distanceAway, 0)
};
this.sceneView.Scene.RootNode.AddChildNode(cubeNodeBelow);
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
public class SphereNode : SCNNode
{
public SphereNode(float size, UIColor color)
{
var rootNode = new SCNNode
{
Geometry = CreateGeometry(size, color),
Position = new SCNVector3(0, size / 2, 0)
};
AddChildNode(rootNode);
}
private static SCNGeometry CreateGeometry(float size, UIColor color)
{
var material = new SCNMaterial();
material.Diffuse.Contents = color;
var geometry = SCNSphere.Create(size);
geometry.Materials = new[] { material };
return geometry;
}
}
}
Next Step : Corner radius
After you have mastered this you should try Corner radius