Environmental texturing
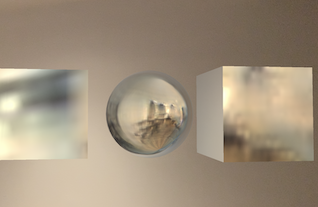
Description
At first, I thought the ability to use reflective materials in Augmented Reality would look really cool.
Unfortunately I was a bit underwhelmed with the initial results by soley manipulating material Metalness
and Roughness
. I partly wonder if this is due to poor lighting conditions in my study.
Though I am led to believe that better results can be achieved using an 'environmental probe', which is something I may try in a future lesson.
Video
No video yet
Code
using ARKit;
using Foundation;
using SceneKit;
using System;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
this.sceneView = new ARSCNView
{
AutoenablesDefaultLighting = true
};
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration
{
AutoFocusEnabled = true,
PlaneDetection = ARPlaneDetection.Horizontal,
EnvironmentTexturing = AREnvironmentTexturing.Automatic,
LightEstimationEnabled = true,
WorldAlignment = ARWorldAlignment.Gravity
}, ARSessionRunOptions.ResetTracking | ARSessionRunOptions.RemoveExistingAnchors);
var sphereGeometry = SCNSphere.Create(0.125F);
sphereGeometry.FirstMaterial.LightingModelName = SCNLightingModel.PhysicallyBased;
sphereGeometry.FirstMaterial.Metalness.Contents = new NSNumber(1.0F);
sphereGeometry.FirstMaterial.Roughness.Contents = new NSNumber(0.0F);
var sphereNode = new SCNNode();
sphereNode.Geometry = sphereGeometry;
this.sceneView.Scene.RootNode.AddChildNode(sphereNode);
var boxGeometry = SCNBox.Create(0.25f, 0.25f, 0.25f, 0f);
boxGeometry.FirstMaterial.LightingModelName = SCNLightingModel.PhysicallyBased;
boxGeometry.FirstMaterial.Metalness.Contents = new NSNumber(1.0F);
boxGeometry.FirstMaterial.Roughness.Contents = new NSNumber(0.0F);
var boxNode = new SCNNode();
boxNode.Position = new SCNVector3(0.3f, 0, 0);
boxNode.Geometry = boxGeometry;
this.sceneView.Scene.RootNode.AddChildNode(boxNode);
var planeGeometry = SCNPlane.Create(0.25f, 0.25f);
planeGeometry.FirstMaterial.LightingModelName = SCNLightingModel.PhysicallyBased;
planeGeometry.FirstMaterial.Metalness.Contents = new NSNumber(1.0F);
planeGeometry.FirstMaterial.Roughness.Contents = new NSNumber(0.0F);
var planeNode = new SCNNode();
planeNode.Position = new SCNVector3(-0.3f, 0, 0);
planeNode.Geometry = planeGeometry;
this.sceneView.Scene.RootNode.AddChildNode(planeNode);
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
}
Next Step : Play a video on a business card
After you have mastered this you should try Play a video on a business card