Node animation
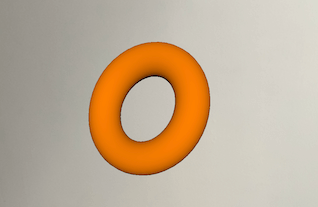
Description
We can animate nodes in a scene using SCNAction
. A number of properties can be animated such as size, position and opacity.
Video
No video yet
Code
using ARKit;
using SceneKit;
using System;
using UIKit;
namespace XamarinArkitSample
{
public partial class ViewController : UIViewController
{
private readonly ARSCNView sceneView;
public ViewController(IntPtr handle) : base(handle)
{
this.sceneView = new ARSCNView
{
AutoenablesDefaultLighting = true
};
this.View.AddSubview(this.sceneView);
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
this.sceneView.Frame = this.View.Frame;
}
public override void ViewDidAppear(bool animated)
{
base.ViewDidAppear(animated);
this.sceneView.Session.Run(new ARWorldTrackingConfiguration
{
AutoFocusEnabled = true,
PlaneDetection = ARPlaneDetection.Horizontal,
LightEstimationEnabled = true,
WorldAlignment = ARWorldAlignment.GravityAndHeading
}, ARSessionRunOptions.ResetTracking | ARSessionRunOptions.RemoveExistingAnchors);
var size = 0.05f;
var torusNode = new TorusNode(size, UIColor.Orange);
var rorateAction = SCNAction.RotateBy((float)(Math.PI), 0, 0, 5.0);
var repeatForever = SCNAction.RepeatActionForever(rorateAction);
torusNode.RunAction(repeatForever);
this.sceneView.Scene.RootNode.AddChildNode(torusNode);
}
public override void ViewDidDisappear(bool animated)
{
base.ViewDidDisappear(animated);
this.sceneView.Session.Pause();
}
public override void DidReceiveMemoryWarning()
{
base.DidReceiveMemoryWarning();
}
}
public class TorusNode : SCNNode
{
public TorusNode(float size, UIColor color)
{
var rootNode = new SCNNode
{
Geometry = CreateGeometry(size, color)
};
AddChildNode(rootNode);
}
private static SCNGeometry CreateGeometry(float size, UIColor color)
{
var material = new SCNMaterial();
material.Diffuse.Contents = color;
var geometry = SCNTorus.Create(size / 2, size / 6);
geometry.Materials = new[] { material };
return geometry;
}
}
}
Next Step : Image recognition
After you have mastered this you should try Image recognition